@gfazioli/mantine-text-animate
A Mantine component that allows you to animate text with various effects. Additionally, it provides other sub components such as TextAnimate.TextTicker, TextAnimate.Typewriter, TextAnimate.NumberTicker, and TextAnimate.Spinner. You can also use three useful hooks: useTextTicker, useTypewriter, and useNumberTicker.
Installation
After installation import package styles at the root of your application:
You can import styles within a layer @layer mantine-text-animate
by importing @gfazioli/mantine-text-animate/styles.layer.css
file.
Usage
The TextAnimate
component allows you to animate text with various effects. The animate
prop controls the initial display and the direction of the animation.
In the example below, try selecting none
to display nothing. With static
, the text will appear static.
Selecting Animate In
will display the entering animation, and with Animate Out
, the exiting animation will be shown.
animateProps
The animateProps
prop allows you to pass additional props to the animation.
by
The by
prop allows you to animate text by the entire text, character by character, per word or line by line.
By using the by="line"
prop, you can animate text line by line.
Styling
Of course, you can use the component with your own styles.
onAnimationStart and onAnimationEnd
You can use the onAnimationStart
and onAnimationEnd
props to handle animation events.
TextAnimate.Typewriter component
The TextAnimate.Typewriter
component allows you to animate text with a typewriter effect.
multiline
By using the multiline
prop, you can animate text line by line.
leftSection
You may use the leftSection
prop to display a section before the text.
>
|Styling
Of course, you can use the component with your own styles.
onTypeEnd and onTypeLoop
You can use the onTypeEnd
and onTypeLoop
props to handle typewriter events.
useTypewriter() hook
The TypeWriter
is build by using the useTypewriter
hook.
Done
The interface of the useTypewriter
hook is the following:
And the return value of the useTypewriter
hook is the following:
multiline with useTypewriter() hook
You may use the multiline
prop with the useTypewriter
hook.
In this case the text
will be an array of strings.
Example: Terminal
Below a funny example of a terminal.
TextAnimate.Spinner component
The TextAnimate.Spinner
component allows you to animate text with a spinner effect.
repeatText and repeatCount
TextAnimate.NumberTicker component
The TextAnimate.NumberTicker
component allows you to animate text with a number ticker effect.
0
useNumberTicker() hook
The TextAnimate.NumberTicker
is built by using the useNumberTicker
hook.
Done
0
The interface of the useNumberTicker
hook is the following:
and the return value of the useNumberTicker
hook is the following:
Example: NumberTicker
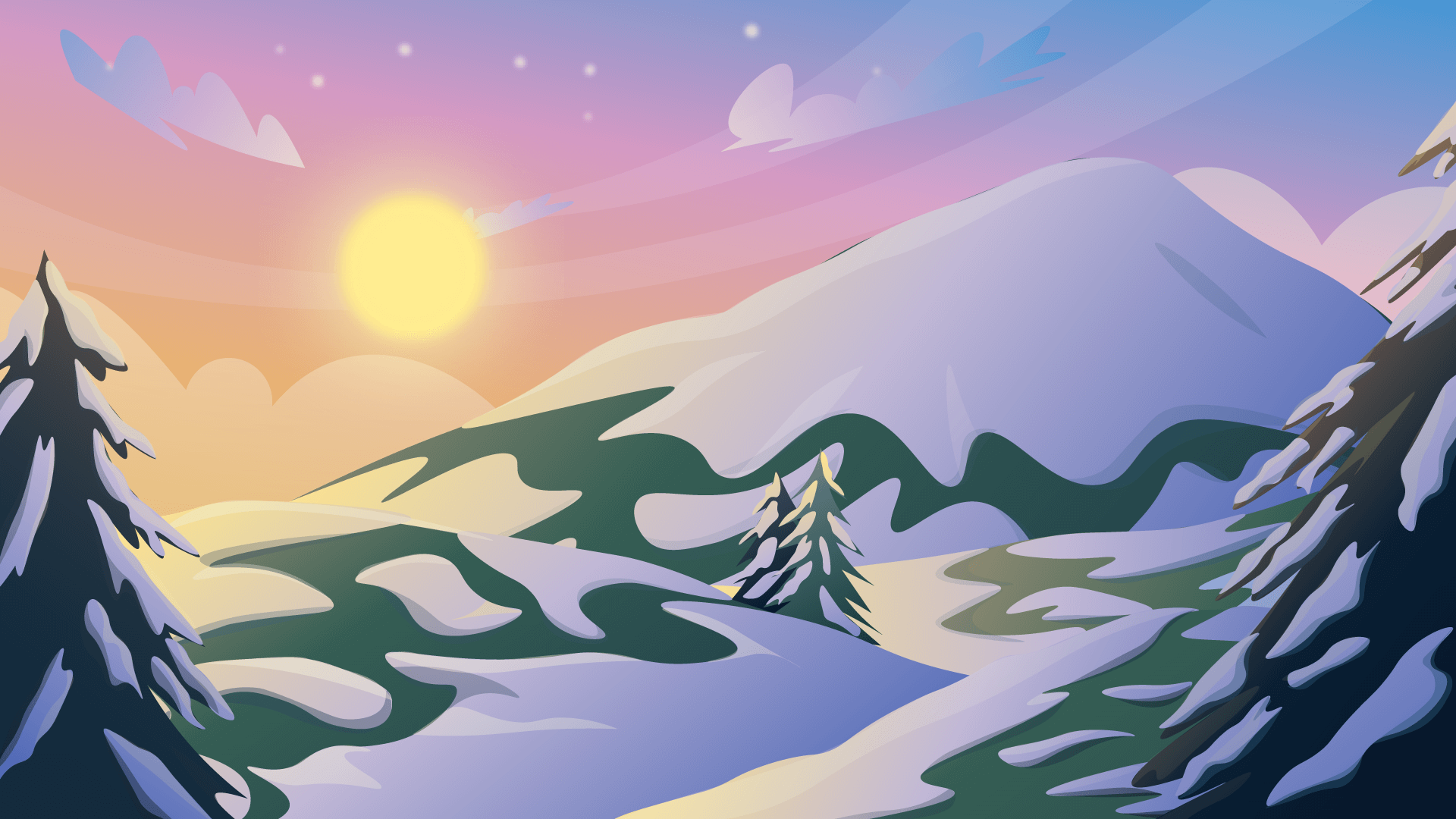
Norway Fjord Adventures
100.99
$With Mountain Expeditions, you can immerse yourself in the breathtaking mountain scenery through tours and activities in and around the majestic peaks
Download0
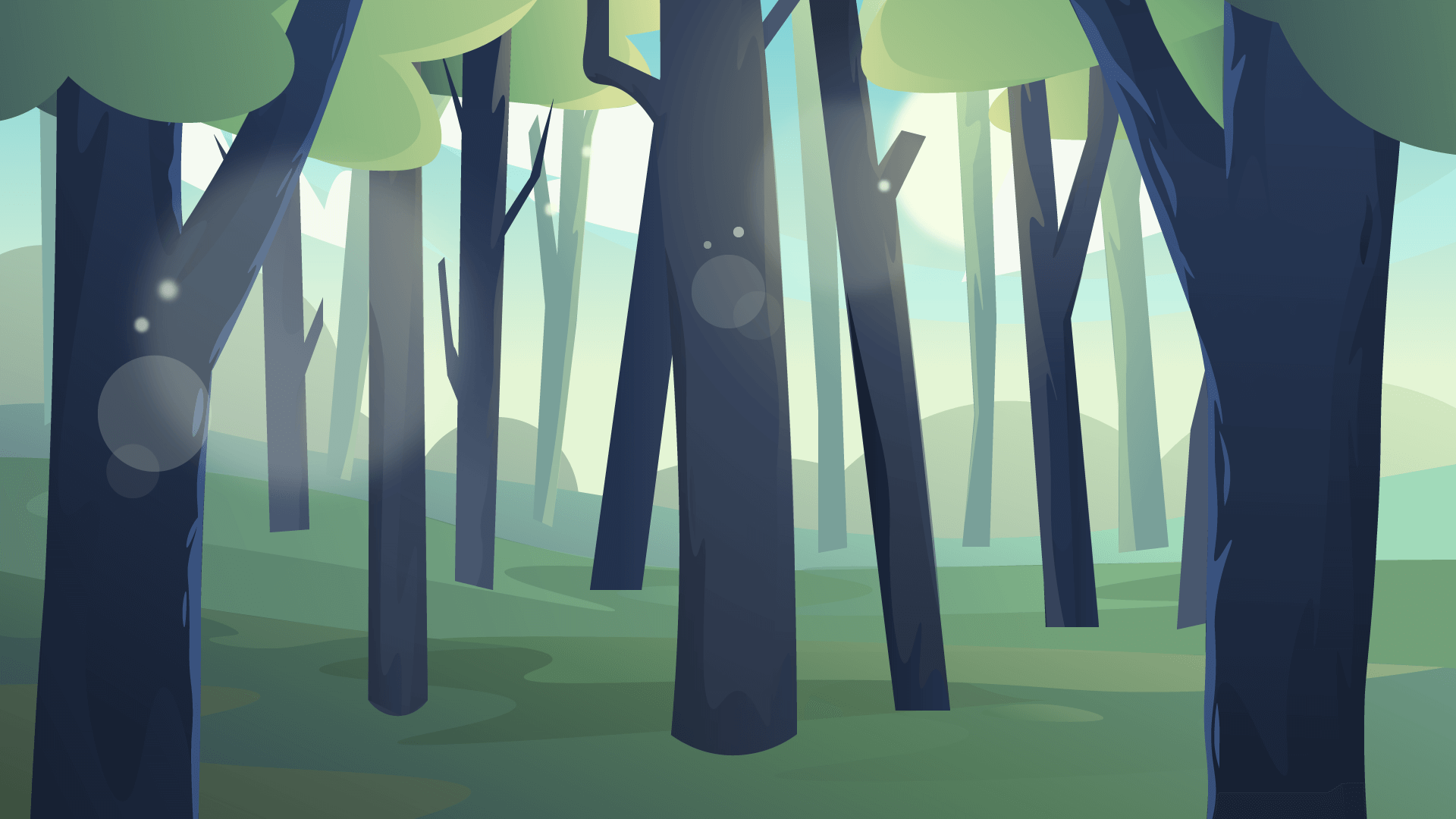
Norway Fjord Adventures
200.00
$With Fjord Tours you can explore more of the magical fjord landscapes with tours and activities on and around the fjords of Norway
Download0
TextAnimate.TextTicker
The TextAnimate.TextTicker
component allows you to animate text with a text ticker effect.
useTextTicker() hook
The TextAnimate.TextTicker
is built by using the useTextTicker
hook.
Done
The interface of the useTextTicker
hook is the following:
and the return value of the useTextTicker
hook is the following: