@gfazioli/mantine-marquee
A Mantine component that allows you to create a marquee effect with a list of elements.
Installation
After installation import package styles at the root of your application:
You can import styles within a layer @layer mantine-marquee
by importing @gfazioli/mantine-marquee/styles.layer.css
file.
Usage
The Marquee
component allows you to create a marquee effect with any content.
Multiple
The Marquee
component allows you to create a marquee effect with multiple items.
Vertical
The Marquee
component allows you to create a marquee effect with multiple items in vertical direction.
Examples
Testimonials
Your customers feedback is important to you. Use Marquee to display your testimonials.
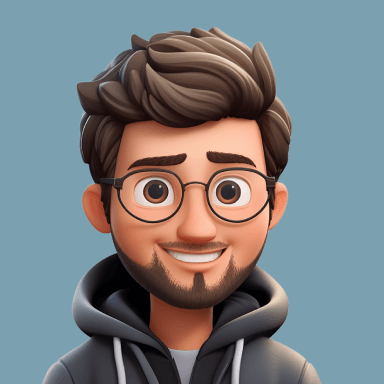
John Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
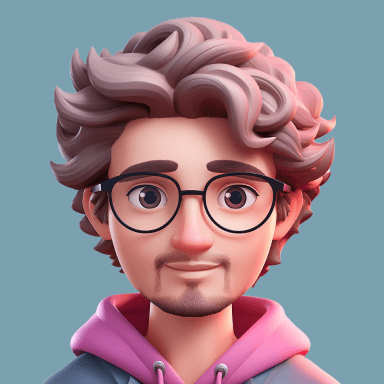
Jane Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
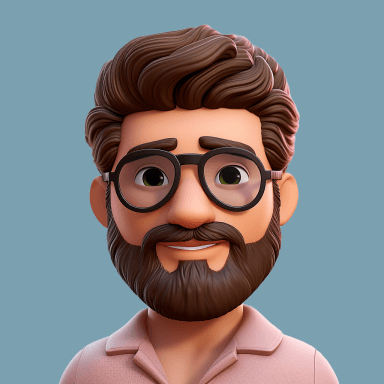
Jessica Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
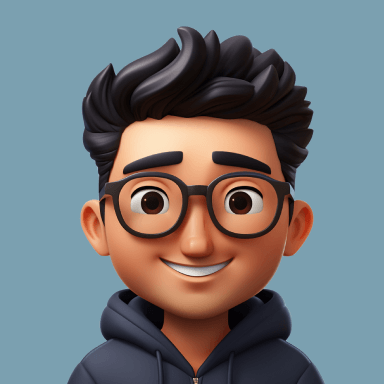
Jack Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
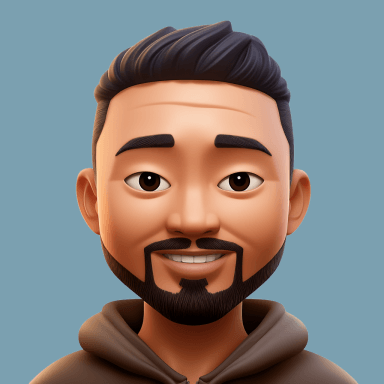
Jill Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
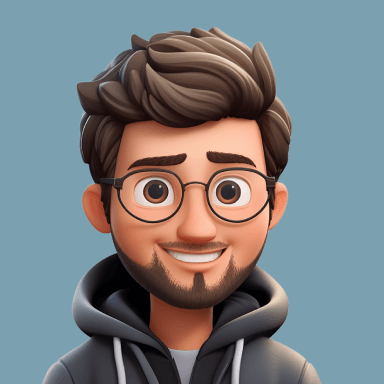
John Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
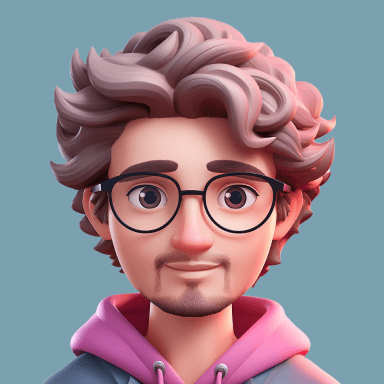
Jane Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
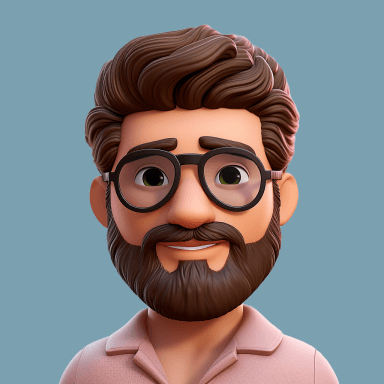
Jessica Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
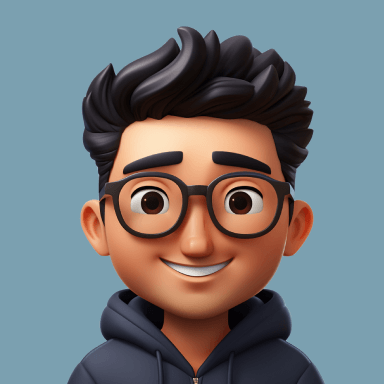
Jack Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
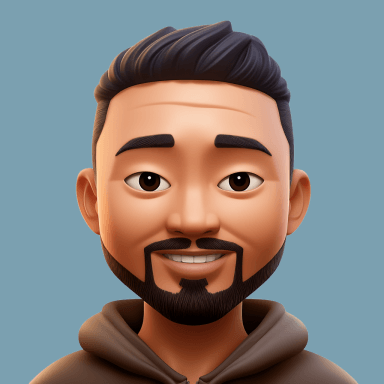
Jill Doe
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
Branding
One of the most common use cases for Marquee
is to create branding banners.