@gfazioli/mantine-onboarding-tour
A Mantine component enables you to create a onboarding-tour effect using overlays, popovers, and onboarding tours, which enhances element visibility and interactivity.
Installation
After installation import package styles at the root of your application:
You can import styles within a layer @layer mantine-onboarding-tour
by importing @gfazioli/mantine-onboarding-tour/styles.layer.css
file.
Example
Here is a full page example of an Onboarding Tour.
Open Onboarding Tour example page
Usage
The OnboardingTour
component allows to create onboarding experiences for your users.
A simple example of the Onboarding Tour component
👉 New amazing Mantine extension component
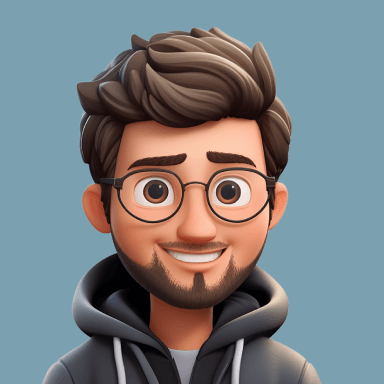
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
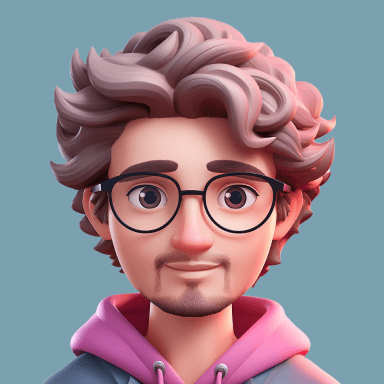
Jane Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
OnboardingTourStep
The OnboardingTourStep
interface defines the structure of each step in the tour.
Both header
, title
, content
, and footer
can be a string, a React component, or a function that receives tourController
and returns a React component.
OnboardingTourController
The OnboardingTourController
interface provides the current state of the tour. It also provides a series of actions to interact with the tour, such as the action to go to the next step nextStep()
or to end the tour endTour()
.
Define the onboarding tour
You can define your onboarding tour by using the OnboardingTourStep
array.
You may also handle the header
, title
, content
, and footer
by using the OnBoardingTour
component props. In this case the OnboardingTourStep
will be ignored.
Anyway, the OnboardingTourStep
are always available in the OnboardingTourController
and you can use them to display any variant. Fo example,
Custom entry
You may use any custom entry in the OnboardingTourStep
list to customize the tour. For example, here we're going to display some extra information in the footer, by using a custom property price
:
Then we can use the footer
prop to display the price
:
Custom Popover Content
You may use the controller to override the default behavior of the tour or to add custom logic to the tour. For example, you could replace the default Popover content with a custom one:
Custom Stepper
The stepper
prop allows you to customize the stepper of the tour. For example, you could use it to display a progress bar or a custom list of steps. Currently, the OnboardingTour
component use the Mantine Stepper component. You can use the stepperProps
and stepperStepProps
props to customize the stepper. Of course, you can build your own using the stepper
prop.
OnboardingTour.Target
You have to use the OnboardingTour.Target
when your tour component are not visible as children of the OnboardingTour
component.
For example, here is an example of a tour component that is not a child of the OnboardingTour
component.
The above example won't work because we can't get the data-onboarding-tour-id
attribute of the AnotherComponent
component.
In this case you have to use the OnboardingTour.Target
component to make it work. In short, instead of using the data-onboarding-tour-id
attribute of the component, you have to wrap your component with OnboardingTour.Target
.
OnboardingTour.FocusReveal
The OnboardingTour.FocusReveal
component allows highlighting and making any component on your page more visible. The highlighting process can be controlled by three main properties:
withOverlay
: displays a dark overlay across the entire page except for the highlighted componentwithReveal
: scrolls the page to make the component to be highlighted visiblefocusEffect
: applies a series of predefined effects when the component is highlighted
Naturally, we have the focused
prop that controls when the component should be highlighted.
Note: In all examples, we use the
onBlur
event to remove focus from the component for demonstration purposes. In a real-world scenario, you might also use thefocused
prop to control the focus state.
A simple example of the Focus Reveal component
👉 Scroll up the page to remove the focus
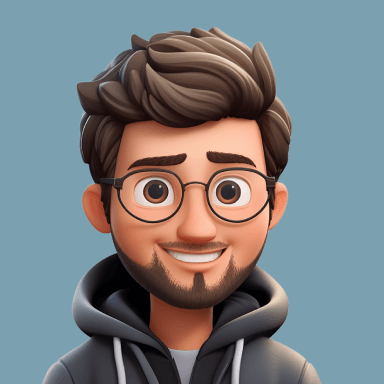
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
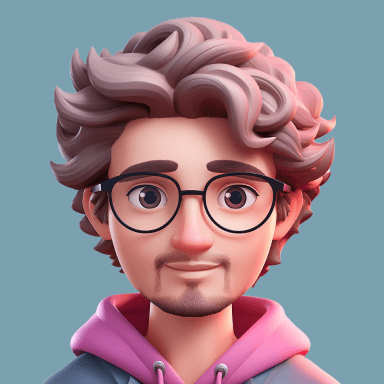
Jane Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
Uncontrolled Mode
The OnboardingTour.FocusReveal
component can also be used in an uncontrolled mode. In this mode, the component will automatically highlight the children.
Simple (uncontrolled) Example
The defaultFocused
props is set to true
, card below is focused by default
👇
Scroll down
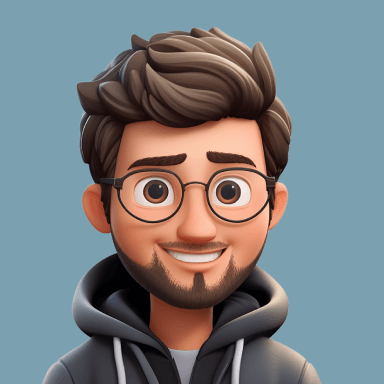
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
OnboardingTour.FocusReveal.Group
If you want to highlight multiple components, you can use the OnboardingTour.FocusReveal.Group
component. This component allows you to highlight multiple components at the same time.
This also allows for controlling multiple OnboardingTour.FocusReveal
components simultaneously, setting some common properties for all the components.
Note: The
OnboardingTour.FocusReveal.Group
component does not have thefocused
prop. Instead, use thefocused
prop in theOnboardingTour.FocusReveal
component. In addition, thewithReveal
prop is set tofalse
by default. ThedefaultFocused
prop is set totrue
by default.
Group Example
The defaultFocused
props is set to true
, card below is focused by default
👇
Scroll down
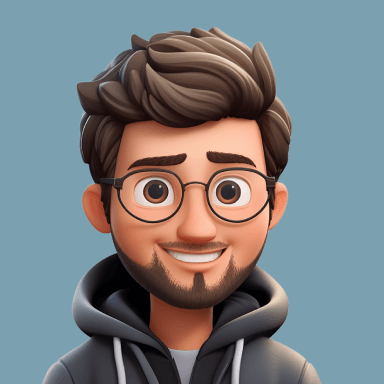
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
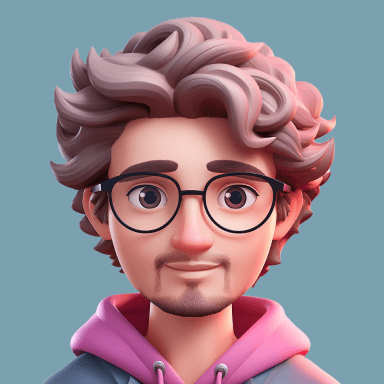
Jane Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
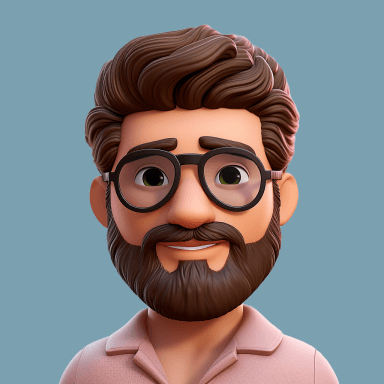
Jessica Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
Below another example of using the OnboardingTour.FocusReveal.Group
component.
Group Example
The defaultFocused
props is set to true
, card below is focused by default
👇
Scroll down
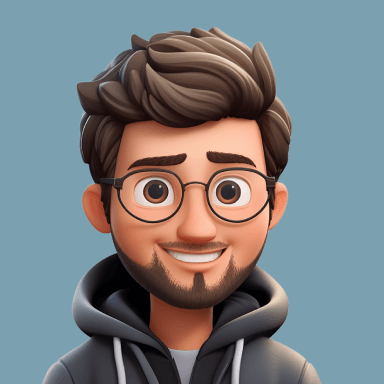
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
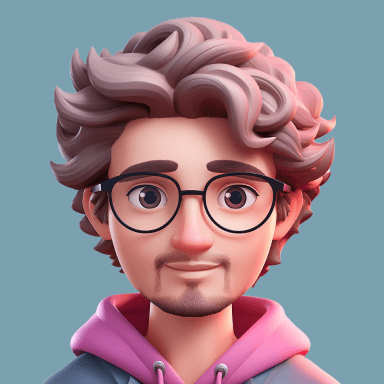
Jane Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
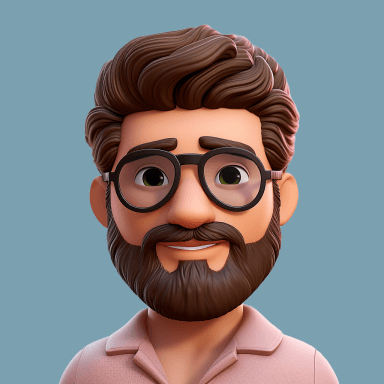
Jessica Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
withReveal
The withReveal
props scrolls the page to make the component to be highlighted visible. This is useful when the component is not visible on the screen. Internally, the component uses the scrollIntoView
method to make the component visible, from the Mantine useScrollIntoView()
hook.
Of course, you can customize the scroll behavior by using the revealProps
prop. This prop accepts the same properties as the useScrollIntoView()
method.
Custom Reveal Props Example
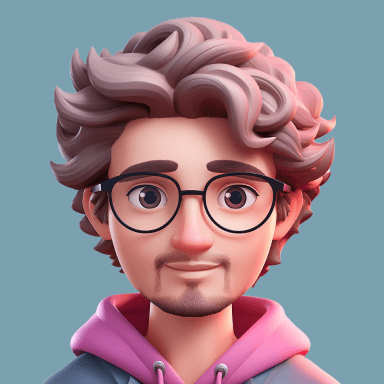
Jane Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
Note: The
onScrollFinish
callback is not available in therevealsProps
prop. Instead, use theonRevealFinish
prop.
withOverlay
The withOverlay
prop displays a dark overlay across the entire page except for the highlighted component. This is useful when you want to focus the user's attention on a specific component. The overlay is customizable by using the overlayProps
prop.
Overlay Example
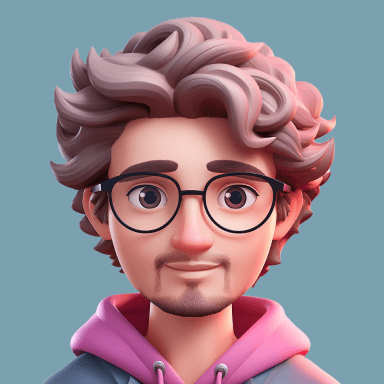
Jane Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
scrollableRef
The scrollableRef
prop allows you to specify a custom scrollable element. This is useful when the component to be highlighted is inside a scrollable container. The scrollableRef
prop accepts a React.RefObject<HTMLElement>
.
With simple Paper container
Paper container Example
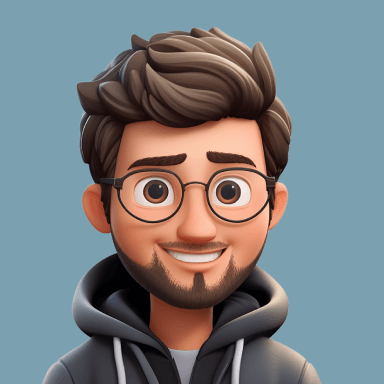
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
Below, by using the ScrollArea
component, we can create a scrollable container.
ScrollArea
Custom Focus Mode
The OnboardingTour.FocusReveal
component allows you to create a custom focus mode. This is useful when you want to create a custom focus effect.
Custom Focused Mode Example
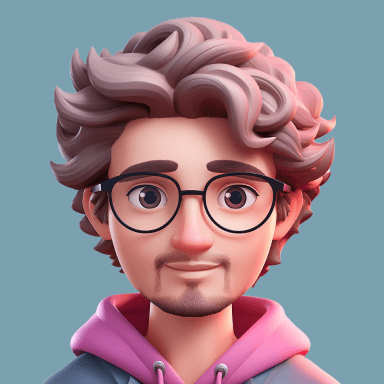
Jane Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
With Popover
In addition to focus and reveal, you can add a Popover
display when the element gains focus by using the popoverContent
prop.
Popover example
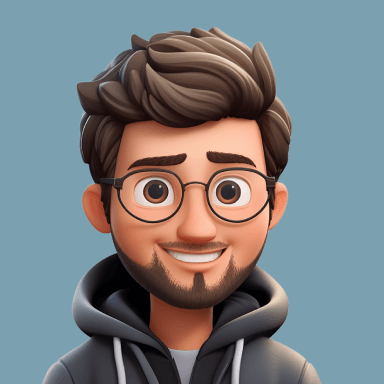
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
You can use the popoverProps
prop (are the same as the Mantine Popover
component. You can find the documentation here) to manipulate the Mantine Popover
component.
Popover example
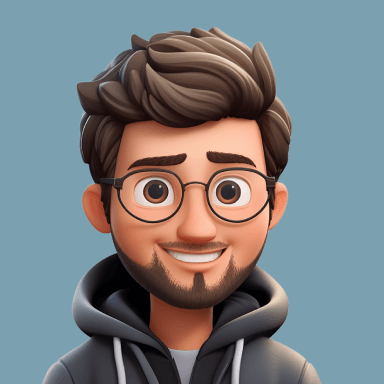
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
Example: Cycle
Here are some examples of how to use the OnboardingTour.FocusReveal
component in different scenarios.
Cycle Example
Use the arrow keys to cycle through the testimonials
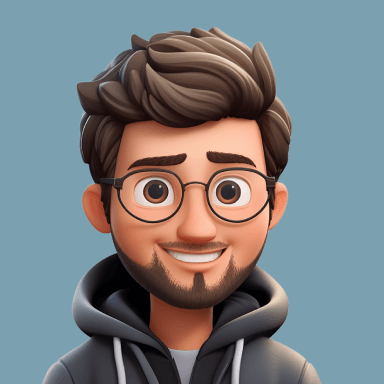
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
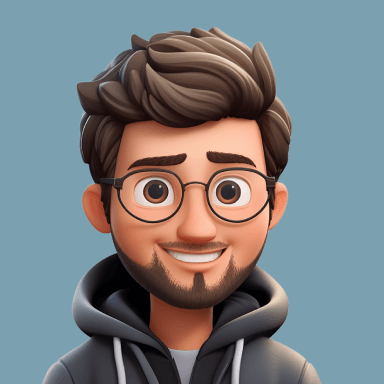
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
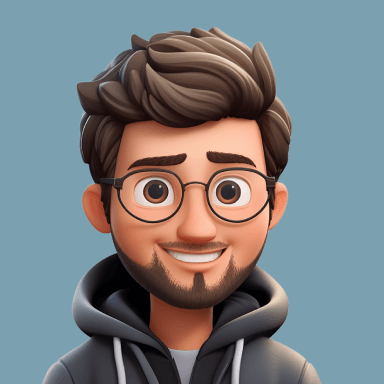
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
Example: Multiple Focus components
Multiple components Example
Use the arrow keys to cycle through the testimonials
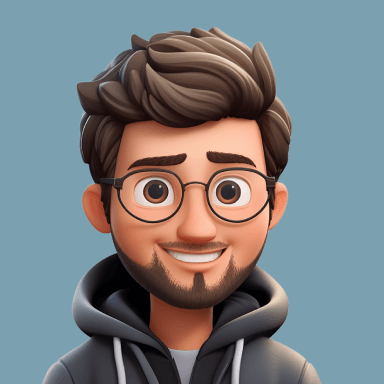
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
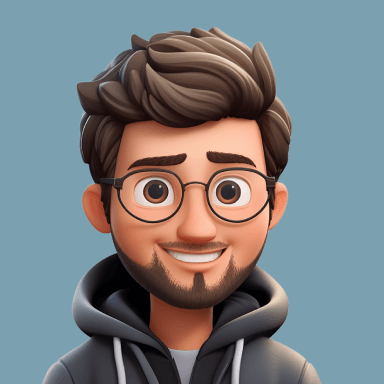
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.
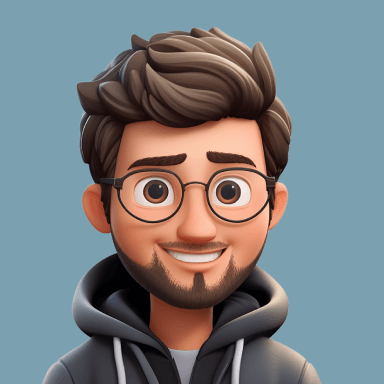
John Doe
Scroll the page 👆up or 👇down to remove the focus from the card. In practice, make this component invisible so that the onBlur event will be triggered.