@gfazioli/mantine-parallax
A Mantine component that allows you to create the famous Apple TV parallax effect.
Installation
After installation import package styles at the root of your application:
You can import styles within a layer @layer mantine-parallax
by importing @gfazioli/mantine-parallax/styles.layer.css
file.
Usage
Despite the Parallax
component allowing you to create the famous Apple TV Card effect, you can use this component to manipulate the perspective and rotation of an element. It is also possible to act on the skew of the element and disable the parallax effect when hovering with the mouse. See Initial values and Skew below.
The disabled
prop allows you to disable the effect. Both perspective
and initialPerspective
props will be disabled if the value is greater or equal to 10000
.
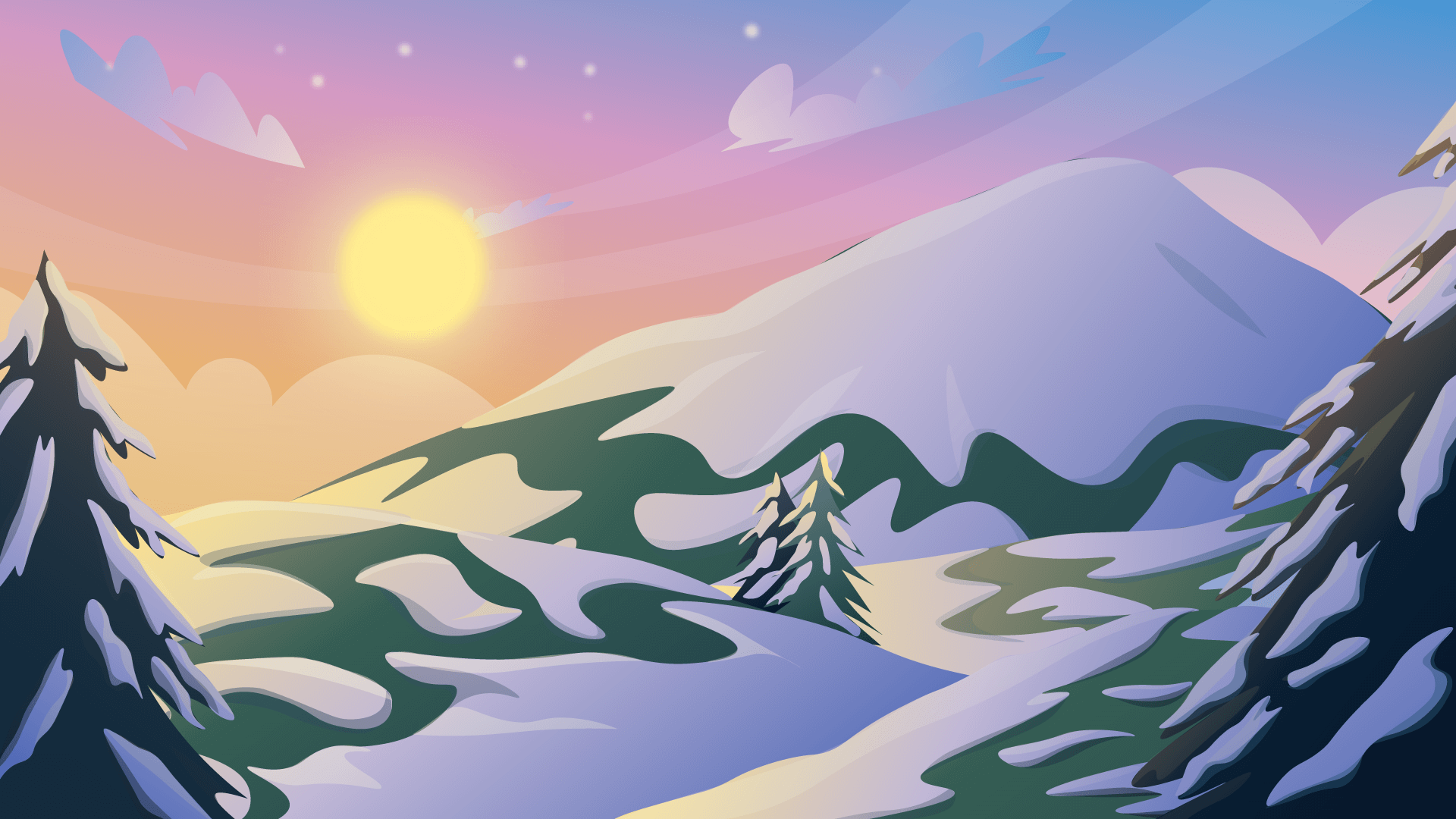
Norway Fjord Adventures
With Fjord Tours you can explore more of the magical fjord landscapes with tours and activities on and around the fjords of Norway
Initial values and Skew
You can set the initial rotation of the card using the initialPerspective
, initialRotationX
, initialRotationY
, and initialRotationZ
props.
You may also use the initialSkewX
and initialSkewY
props to set the initial skew of the card.
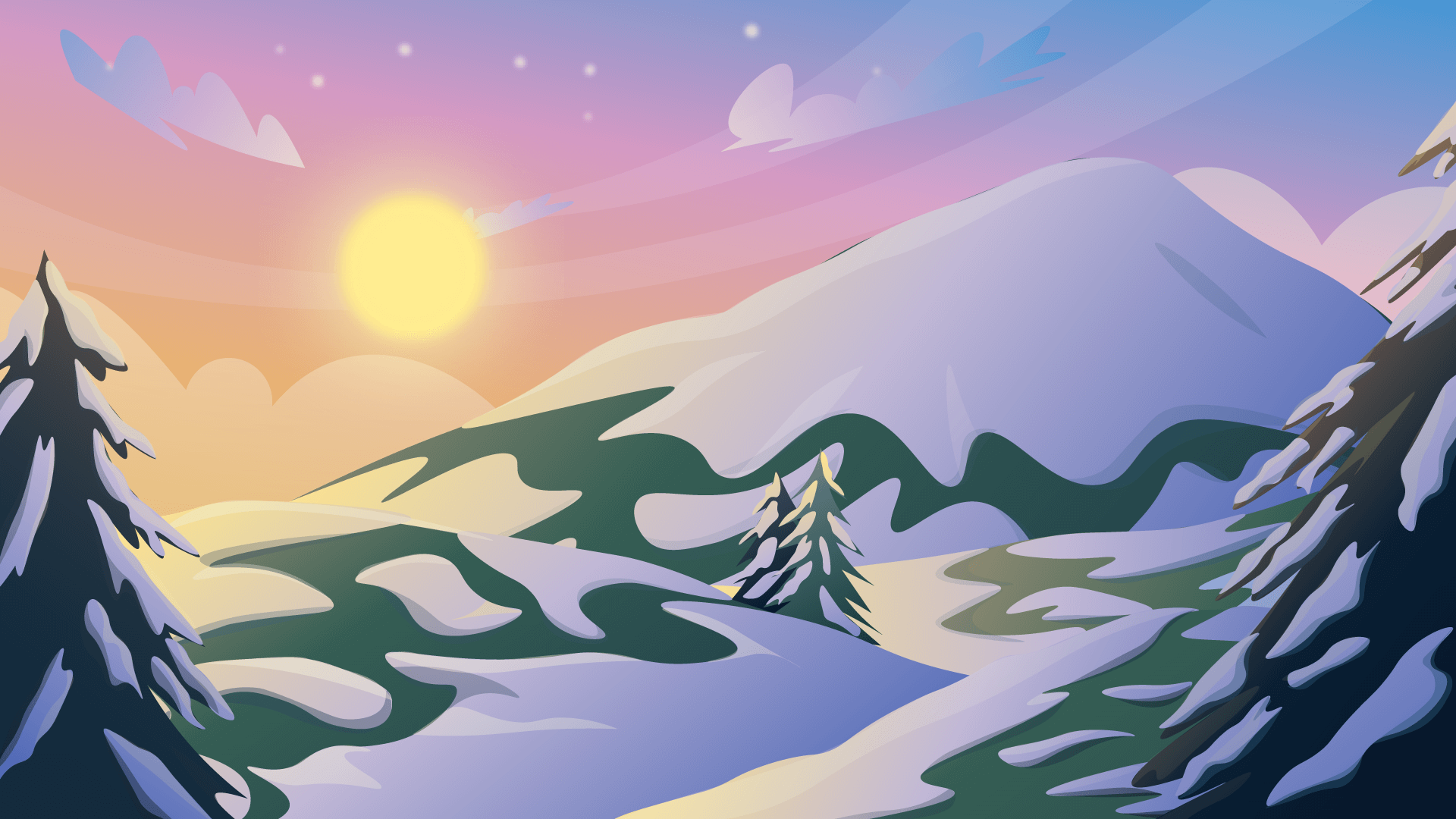
Norway Fjord Adventures
With Fjord Tours you can explore more of the magical fjord landscapes with tours and activities on and around the fjords of Norway
Scroll
The initial values of the Parallax
component can be set based on the scroll position. You can use the useWindowScroll()
hook to get the scroll position and pass it to the Parallax
component.
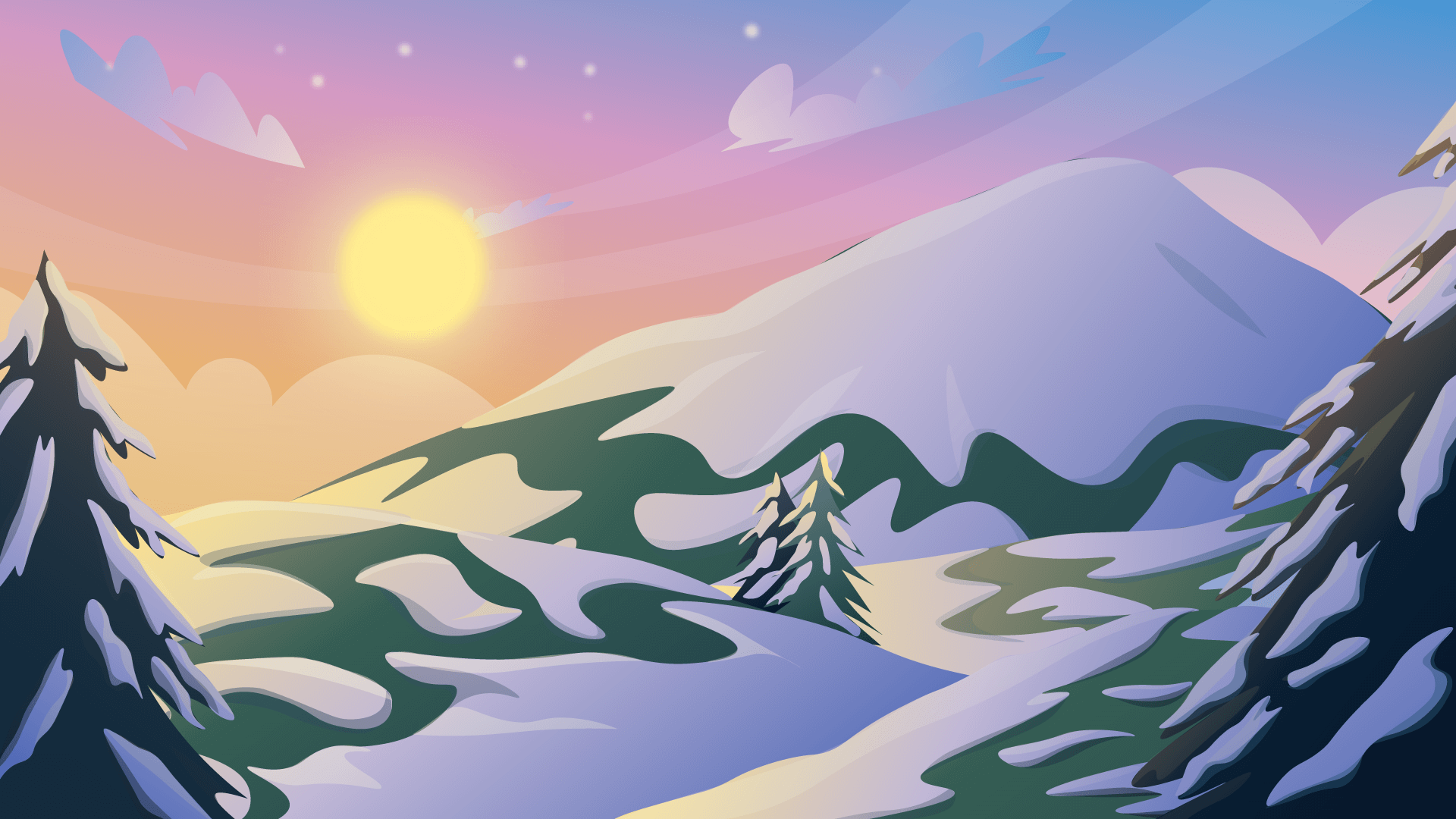
Norway Fjord Adventures
With Fjord Tours you can explore more of the magical fjord landscapes with tours and activities on and around the fjords of Norway
Simple usage
Below a super simple example of how to use the Parallax
component.
Parallax
Amazing contentParallax effect component. Hover to see the effect.
Parallax Effects
In addition to the classic Apple TV Card effect, you can use the Parallax
component to create a parallax effects both for the background and for the content. You may use the backgroundParallax
and backgroundParallaxThreshold
props to enable and manage the parallax effect.
Background
Here is an example of the parallax effect used for the background.
Content
Of course, you can also use the parallax effect for the content. You may use the contentParallax
and contentParallaxDistance
props to enable and manage the parallax effect.
Parallax
Amazing contentParallax effect component. Hover to see the effect.
Example: Complex
Below a more complex example of how to use the Parallax
component.
Parallax
Amazing contentParallax effect component. Hover to see the effect.
Example: Shadow
Give free rein to your creativity. You can use the Parallax
component to create shading effects.
Parallax
Amazing parallax effect component. Hover to see the effect.